Hey Everyone today we will see how to implement WordPress Ajax Login And Register Without A Plugin. I will give a full step-by-step tutorial and the code.
Ajax Login system improves our user experience by without refreshing the current page by utilizing PHP & javascript. For implementing the Ajax login system without a plugin we need to install a child theme and some files and codes into it.
What is Ajax Login
Ajax Login is method by which user will get a popup or have to fill the form to login and this method don’t refreshes the website and allow to run the login process in background. This feature improves our login and user experience.
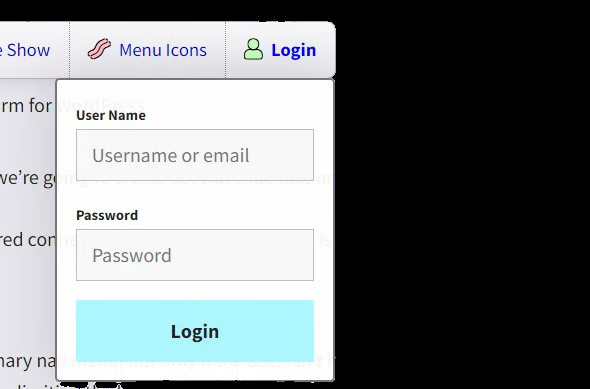
Step-by-step guide to install ajax login without plugin
Step 1: Add a custom folder name wpt-ajax-login
to your child theme and create the following files in it.
- wpt-ajax-login.css
- wpt-ajax-login.js
- login-form.php
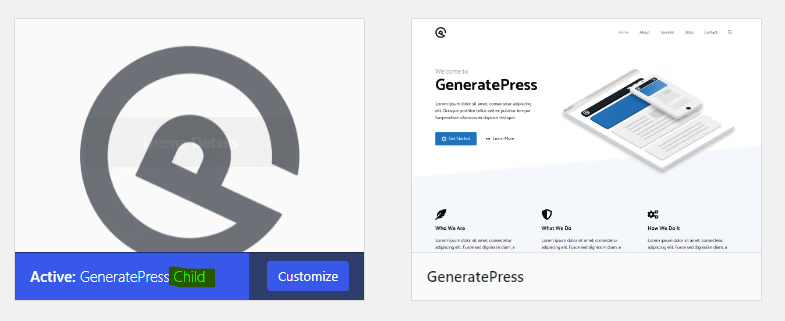
Step 2: Add the below HTML code into login-form.php
file
<?php
defined('WPINC') || die();
?>
<p class="form-row">
<label for="wptajl-username"><?php esc_html_e('User Name', 'wp-tutorials'); ?></label>
<input id="wptajl-username" name="username" type="text" placeholder="Username or email" />
</p>
<p class="form-row">
<label for="wptajl-password"><?php esc_html_e('Password', 'wp-tutorials'); ?></label>
<input id="wptajl-password" name="password" type="password" placeholder="Password" />
</p>
<p class="form-row">
<button class="wptajl-login button"><?php esc_html_e('Login', 'wp-tutorials'); ?></button>
</p>
Step 3: Make a new file name wpt-ajax-login.php
in your main theme directory for the back-end logic purpose. Add the below code in this file.
Read more: WordPress Breadcrumbs Without Plugin
<?php
defined('WPINC') || die();
const WPTAJL_MENU_LOCATION = 'primary';
const WPTAJL_LOGIN_RETRY_PAUSE = 5; // seconds between retries
const WPTAJL_SPINNER_FILE_NAME = 'tail-spin.svg';
const WPTAJL_LOGIN_ACTION = 'wptajl-login';
const WPTAJL_LOGIN_FORM_FILE_NAME = 'login-form.php';
function wptajl_init() {
add_action('wp_ajax_nopriv_' . WPTAJL_LOGIN_ACTION, 'wptajl_try_to_login');
}
add_action('init', 'wptajl_init');
function wptajl_client_ip() {
if (!empty($_SERVER['HTTP_CLIENT_IP'])) {
return filter_var($_SERVER['HTTP_CLIENT_IP'], FILTER_VALIDATE_IP);
} elseif (!empty($_SERVER['HTTP_X_FORWARDED_FOR'])) {
return filter_var($_SERVER['HTTP_X_FORWARDED_FOR'], FILTER_VALIDATE_IP);
} else {
return filter_var($_SERVER['REMOTE_ADDR'], FILTER_VALIDATE_IP);
}
}
function wptajl_enqueue_scripts() {
$base_uri = get_stylesheet_directory_uri();
$version = wp_get_theme()->get('Version');
wp_enqueue_style('wptajl', $base_uri . '/wpt-ajax-login/wpt-ajax-login.css', null, $version);
wp_enqueue_script('wptajl', $base_uri . '/wpt-ajax-login/wpt-ajax-login.js', array('jquery'), $version, true);
wp_localize_script('wptajl', 'wptajlData', array(
'ajaxUrl' => admin_url('admin-ajax.php'),
'action' => WPTAJL_LOGIN_ACTION,
'spinnerUrl' => $base_uri . '/wpt-ajax-login/' . WPTAJL_SPINNER_FILE_NAME,
));
}
add_action('wp_enqueue_scripts', 'wptajl_enqueue_scripts');
function wptajl_try_to_login() {
$client_ip = wptajl_client_ip();
$client_key = 'login_attempt_' . $client_ip;
$response = array('isLoggedIn' => false, 'errorMessage' => '');
if (get_transient($client_key)) {
$response['errorMessage'] = 'Slow down a bit';
} elseif (empty($_POST['username']) || empty($_POST['password'])) {
$response['errorMessage'] = 'Missing username or password';
} else {
$user = wp_authenticate(sanitize_text_field($_POST['username']), sanitize_text_field($_POST['password']));
if (is_a($user, 'WP_User')) {
wp_set_auth_cookie($user->ID, false);
$response['isLoggedIn'] = true;
} else {
$response['errorMessage'] = 'Invalid login';
set_transient($client_key, true, WPTAJL_LOGIN_RETRY_PAUSE);
}
}
wp_send_json($response);
}
Step 5: For Styling our Ajax Login form let’s add the below CSS code.
.sub-menu.wptajl-container {
padding: 1em;
box-shadow: 0 0 3em #00000022;
min-width: 15em;
position: absolute;
}
.wptajl-container input,
.wptajl-container button {
width: 100%;
display: block;
margin-bottom: 1em;
transition: 0.3s;
}
.login-spinner {
display: none;
width: 1.5em;
}
.wptajl-container.working .login-spinner {
display: block;
}
Step 6: To make our code functionable let’s add the below javascript code.
(function($) {
'use strict';
$(window).on('load', function() {
if (typeof wptajlData !== 'undefined') {
$('.wptajl-login.button').click(function(event) {
event.preventDefault();
var container = $(this).closest('.wptajl-container');
tryToLogin(container);
});
$('.wptajl-container input').keypress(function(event) {
if (event.which == 13) {
event.preventDefault();
var container = $(this).closest('.wptajl-container');
tryToLogin(container);
}
});
function tryToLogin(container) {
var request = {
action: wptajlData.action,
username: $(container).find('#wptajl-username').val(),
password: $(container).find('#wptajl-password').val()
};
if (request.username && request.password && !container.hasClass('working')) {
$(container).addClass('working').find('input, button').prop('disabled', true);
$.post(wptajlData.ajaxUrl, request).done(function(response) {
if (response.isLoggedIn) {
location.reload();
} else {
$(container).removeClass('working').find('input, button').prop('disabled', false);
alert(response.errorMessage);
}
});
}
}
}
});
})(jQuery);
Step 7: Finally we have to modify our functions.php file of our child theme to load this login system.
// Include Ajax login code
require_once get_stylesheet_directory() . '/wpt-ajax-login.php';
Step 8: ( Optional ) Brute Force Protection,To prevent brute force attacks, login attempts are rate-limited to one attempt per 5 seconds by using WordPress transients.
Now, users can log into our website without leaving the current page, with a responsive and secure Ajax login system.
Conclusion
In this post we have learned and implemented a Ajax login system which improves user experience by making login form or popup and by not loading and refreshing the page. Hope you found this tutorial helpful if you have encountered any error then let me know in the comment section I will try to assist you.Don’t forget to share and rate this post. Thanks For Reading.
FAQs
How do I create a custom login in WordPress?
You can create a custom login in WordPress by editing the functions.php file or using a custom page template to style the login form without plugins.
Can I create a form in WordPress without a plugin?
Yes, you can create forms in WordPress without plugins by using custom HTML, PHP, and AJAX code in your theme’s files.
How do I add a registration form to WordPress for free?
You can add a free registration form in WordPress by modifying the theme’s functions.php file or using free form-building plugins like WPForms.
How do I make a WordPress login only?
To restrict access to WordPress and make it login-only, use custom code to redirect non-logged-in users to the login page.
How can I create an AJAX search in WordPress without a plugin?
You can implement AJAX search in WordPress by adding custom JavaScript and PHP code that handles the search query without reloading the page.